Example of reading bytes from a FileInputStream Consider a text file example1.txt containing the following: B C
We can write a program to display the numeric value of each byte in this file. The output of the file is as follows: 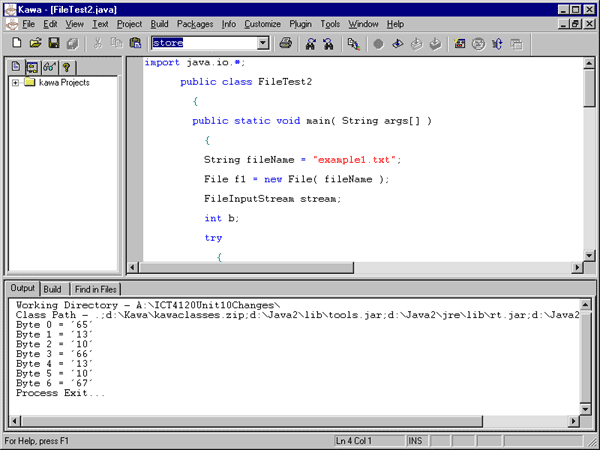
The bytes with values 13 and 10 correspond the characters to carriage return ( <cr> ) and new-line ( <nl> ) respectively (which are how the Windows system indicates a new line in a text file). Java actually views this file as a stream of bytes as follows: A | <cr> | <nl> | B | <cr> | <nl> | C | |
The listing of the application to generate this output is as follows: public class FileTest2 { public static void main( String args[] ) { String fileName = "example.txt"; File f1 = new File( fileName ); FileInputStream stream; int b; try { stream = new FileInputStream(f1); for( int i = 0; i < f1.length(); i++) { // get next byte try { b = stream.read(); // display byte with message on screen System.out.println("Byte " + i + " = " + b + ""); } catch (IOException e) {} } } catch (FileNotFoundException fileException) {} } } // class
Ignore the try and catch statements for now these are related to dealing with exceptional conditions, such as the file not being found. Exceptions, file related and otherwise, are covered later in this unit. This application is given to illustrate how stream , an object of the FileInputStream Back to top 
RITSEC - Global Campus Copyright ?1999 RITSEC- Middlesex University. All rights reserved. webmaster@globalcampus.com.eg |